Submission format
The submission should be a function that, given the data acquisitions for a user in a period of 6 weeks and other user info (Sociodemo and questionnaires), returns a prediction of adherence for the forthcoming 3 scheduled data acquisitions (0 if LOW, 1 if HIGH).
API for Python
def classifier (EQ5D3L_input, Sociodemo_input, SPQ_input, UCLA_input, UTAUT_input, brain_games_input, digital_phenotyping_input, fingertapping_input, mindfulness_input, physical_activity_input, window_start):
return 0
API for Matlab
function result = classifier(EQ5D3L_input, Sociodemo_input, SPQ_input, UCLA_input, UTAUT_input, brain_games_input, digital_phenotyping_input, fingertapping_input, mindfulness_input, physical_activity_input, window_start)
Each input variable is a matrix with the data from the corresponding dataset for a user (using all the columns from the API except for id which should be discarded). The last input (window_start) corresponds to the starting date of the 6 weeks of data acquisition considered.
For the datasets relating to activity data (brain_games, digital_phenotyping, fingertapping, mindfulness and physical_activity) the data provided spans 6 weeks.
Output should be a single value (0 or 1): 0 if predicted adherence for next 1.5 weeks (3 sessions) is LOW or 1 if predicted adherence is HIGH.
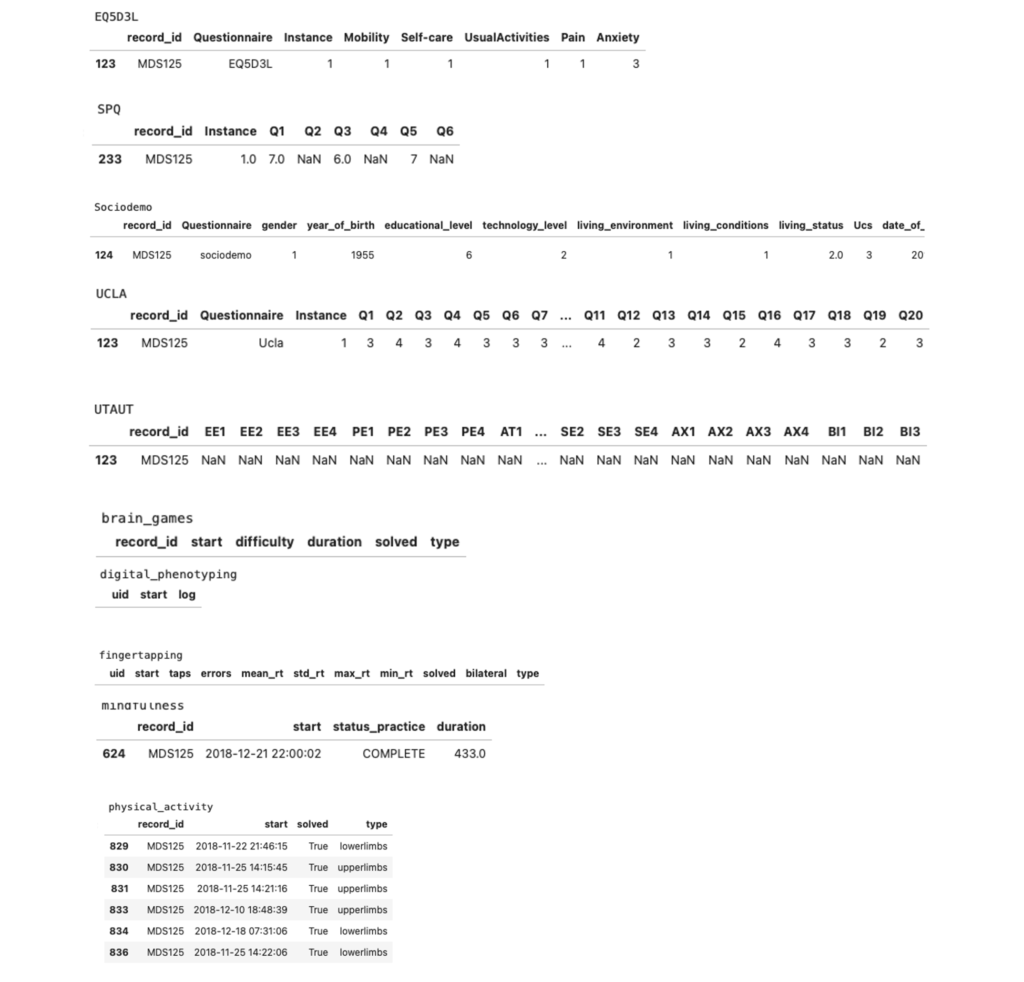
Input arguments for Python
EQ5D3L_input = [[‘MDS125’, ‘EQ5D3L’, 1, 1, 1, 1, 1, 3]]
Sociodemo_input = [[‘MDS125’, ‘sociodemo’, 1, 1955, 6, 2, 1, 1, 2.0, 3, ‘2018-07-04’, ‘2021-05-01’, ‘Dedicated tablet’, ‘Dropout’]]
SPQ_input = [[‘MDS125’, 1.0, 7.0, nan, 6.0, nan, 7, nan]]
UCLA_input = [[‘MDS125’, ‘Ucla’, 1, 3, 4, 3, 4, 3, 3, 3, 3, 3, 2, 4, 2, 3, 3, 2, 4, 3, 3, 2, 3]]
UTAUT_input = [[‘MDS125’, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]
brain_games_input = []
digital_phenotyping_input = []
fingertapping_input = []
mindfulness_input = [[‘MDS125’, ‘2018-12-21 22:00:02’, ‘COMPLETE’, 433.0]]
physical_activity_input = [[‘MDS125’, ‘2018-11-22 21:46:15’, True, ‘lowerlimbs’],
[‘MDS125’, ‘2018-11-25 14:15:45’, True, ‘upperlimbs’],
[‘MDS125’, ‘2018-11-25 14:21:16’, True, ‘upperlimbs’],
[‘MDS125’, ‘2018-12-10 18:48:39’, True, ‘upperlimbs’],
[‘MDS125’, ‘2018-12-18 07:31:06’, True, ‘lowerlimbs’],
[‘MDS125’, ‘2018-11-25 14:22:06’, True, ‘lowerlimbs’]]
result = classifier (EQ5D3L_input, Sociodemo_input, SPQ_input, UCLA_input, UTAUT_input, brain_games_input, digital_phenotyping_input, fingertapping_input, mindfulness_input, physical_activity_input, ‘2018-11-19’)
Input arguments for Matlab
EQ5D3L_input = [{‘MDS125’, ‘EQ5D3L’, [1, 1, 1, 1, 1, 3]}]
Sociodemo_input = [{‘MDS125’, ‘sociodemo’, [1, 1955, 6, 2, 1, 1, 2.0, 3], ‘2018-07-04’, ‘2021-05-01’, ‘Dedicated tablet’, ‘Dropout’}]
SPQ_input = [{‘MDS125’, [1.0, 7.0, nan, 6.0, nan, 7, nan]}]
UCLA_input = [{‘MDS125’, ‘Ucla’, [1, 3, 4, 3, 4, 3, 3, 3, 3, 3, 2, 4, 2, 3, 3, 2, 4, 3, 3, 2, 3]}]
UTAUT_input = [{‘MDS125’, [nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan]}]
brain_games_input = [] %Empty example
In case there is data, it follows the following structure (see DB):
% an array of cell array; each cell array is composed by:
% UID – string
% start – string
% difficulty – string
% array 1×1 float [duration]
% solved – string
% type – string
digital_phenotyping_input = []%Empty example
In case there is data, it follows the following structure (see DB):
% an array of cell array; each cell array is composed by:
% UID – string
% start – string
% log – string
fingertapping_input = []%Empty example
In case there is data, it follows the following structure (see DB):
% an array of cell array; each cell array is composed by:
% UID – string
% start – string
% array 1×6 floats [taps, errors, mean_rt, std_rt, max_rt, min_rt]
% solved – string
% bilateral – string
% type – string
mindfulness_input = [{‘MDS125’, ‘2018-12-21 22:00:02’, ‘COMPLETE’, 433.0}]
physical_activity_input = [{‘MDS125’, ‘2018-11-22 21:46:15’, ‘True’, ‘lowerlimbs’};
{‘MDS125’, ‘2018-11-25 14:15:45’,‘True’, ‘upperlimbs’};
{‘MDS125’, ‘2018-11-25 14:21:16’, ‘True’, ‘upperlimbs’};
{‘MDS125’, ‘2018-12-10 18:48:39’, ‘True’, ‘upperlimbs’};
{‘MDS125’, ‘2018-12-18 07:31:06’, ‘True’, ‘lowerlimbs’};
{‘MDS125’, ‘2018-11-25 14:22:06’, ‘True’, ‘lowerlimbs’}]
result = classifier (EQ5D3L_input, Sociodemo_input, SPQ_input, UCLA_input, UTAUT_input, brain_games_input, digital_phenotyping_input, fingertapping_input, mindfulness_input, physical_activity_input, ‘2018-11-19’);
True output should be 0 (LOW adherence).
# Example inputs for the previously empty examples:
brain_games_input = [ {‘MDS125’, ‘2018-12-18 07:31:06’, [27.00], ‘True’, ‘pairs’} ;
{‘MDS125’, ‘2018-12-18 08:35:00’, [17.00], ‘True’ , ‘ puzzle’} ]
digital_phenotyping_input = [ {‘MDS125’, ‘2018-12-18 07:31:06’, ‘¬øC√≥mo_est√°s?/No_answer’} ;
{‘MDS125’, ‘2018-12-18 07:31:06’, ‘Launcher/Go_to_Divi√©rtete’}]